How to Handle Popups in Selenium WebDriver?
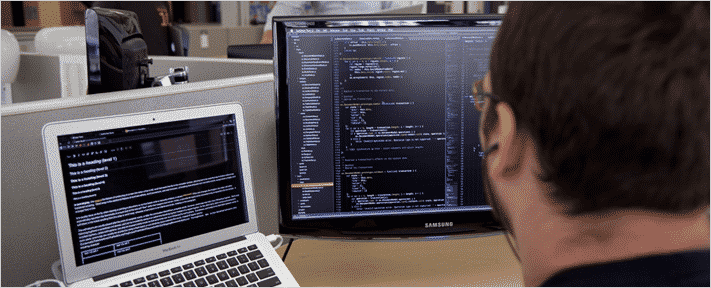
- May 2, 2014
- admin
Creating scalable automated scripts is not easy. Whether you are automating 10 or 1,000 scenarios, you must take the right approach if you want things to work out in your favor. Before you even use scalable automation services, you must take a look at the situation and come up with a strategy that will help you achieve the right results.
Nowadays, more and more engineers are going for automated performance services, all because this helps them deliver results faster and more efficiently. How do you start creating the best scalable automated scripts? Here are some practices that you should use.
- Do Frequent Runs
When it comes to automation, you must make sure you do it as frequently as possible. Obviously, this will require having the right automation tool and setup, as it will allow you to perform several execution cycles instead of fewer ones.
When you have large suites, parallel execution can be set up. This will make the cycle shorter but will allow broader coverage simultaneously. By running frequently, you will ensure that you always meet the quality demands in every single aspect.
- Find Out What to Automate
Establishing what must be automated for performance can be a huge challenge. Automating too little or too much can have terrible effects on your project.
For instance, if you automate too much, then you’ll need a big budget for maintaining scripts without getting any value in return. Meanwhile, if you automate too little, the return on investment will be too low, especially as you don’t spend enough time learning and doing initial setups.
Make sure to identify what must be automated in advance by calculating the ROI.
- Make Sure the Environment Is Right
Another thing that you must pay attention to is selecting the right environment. Ideally, it should be consistent and reliable. You should come up with a strategy that allows you to manage data while taking care of dependencies.
- Look for a Suitable Tool
Not all automation tools can fulfill your needs. It takes some time to find the perfect one, but it’s worth it in the long run. Do some research based on what your wishes are and hunt for the best tool for your project. By doing so, you will be able to execute flows accordingly and use the best practices.
- Build Isolated and Reusable Automation Flows
Building flows is also something you must keep in mind when you want scalable automated scripts. Consider creating isolated flows and think about the way you build your manual cases. You also need to create reusable flows and naming conventions.
- Parameterize Data
Scalable scripts often involve the execution of the same scenario with different sets of data. To achieve this flexibility and avoid duplicating scripts for each data variation, parameterize your data. This allows you to run with different inputs without modifying the underlying structure.
Parameterization enhances script reusability and maintenance. It also facilitates the execution of various scenarios with diverse values, contributing to a more comprehensive coverage.
- Continuous Integration and Continuous Execution
Integrate your automated scripts into a continuous integration (CI) pipeline to ensure continuous execution throughout the development lifecycle. By automating the execution triggered by code changes, you can identify issues early in the process.
Continuous execution supports the agile model and accelerates the feedback loop, allowing teams to address defects promptly. Incorporating scalable automation into CI practices enhances the overall efficiency and reliability of the delivery pipeline.
- Collaboration and Documentation
Effective collaboration between developers, engineers, and other stakeholders is crucial for successful automation, especially when aiming for scalability. Documenting your scripts, including the purpose, expected outcomes, and any specific configurations, promotes transparency and shared understanding.
Maintain detailed documentation on the environment setup, tool configurations, and any dependencies to ensure that the team can easily replicate and extend the automated suite.
- Monitor and Analyze Results
Implement monitoring mechanisms to keep track of execution results. This includes not only identifying failures but also monitoring performance metrics and key indicators. Establishing a robust reporting mechanism allows for quick identification and resolution of issues.
Regularly analyze results to identify patterns, trends, and potential areas for improvement. Continuous evaluation of outcomes contributes to the refinement of scripts and overall strategy, enhancing scalability.
- Scalability Planning
Consider scalability from the initial stages of automation planning. Anticipate future growth and changes in your application and design your automated scripts to accommodate evolving requirements. Scalability planning involves choosing frameworks and practices that can easily adapt to increased volumes and complexity.
By incorporating scalability considerations into your strategy, you can build a resilient framework that evolves alongside your application’s development and ensures long-term efficiency.
Final Thoughts
As you can see, obtaining scalable automated scripts is not as easy as you imagine. However, with the right practices, you can get there in no time. Follow the tips above for the best results.
In the dynamic landscape of software development, the journey to scalable automated scripts is undoubtedly challenging. It requires a continuous commitment to refining strategies, staying abreast of technological advancements, and adapting to the evolving needs of your projects. Embrace a culture of continuous improvement within your practices, encouraging collaboration and knowledge sharing among your teams. Explore emerging automation tools, frameworks, and methodologies that align with your project goals.
Remember that scalability is not a one-time achievement but an ongoing process. Regularly revisit and reassess your strategy to ensure it remains aligned with the changing requirements of your applications. As you encounter new challenges, consider them as opportunities to enhance and optimize your automation framework further. By fostering a mindset of adaptability and continuous learning, you can navigate the complexities of automation and ultimately attain scalable scripts that contribute to the success of your development endeavors.